mirror of
https://github.com/inretio/WordPress-Plugin-Boilerplate
synced 2025-03-03 13:52:49 +02:00
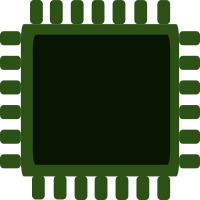
We assume that someone defining WP_LANG_DIR will do so without a trailing slash, since we then add our own before adding in the rest of the path to the .mo file. This change gets removes that assumption by removing our own slash from the string, and wrapping WP_LANG_DIR in trailingslashit() instead.
279 lines
7.3 KiB
PHP
279 lines
7.3 KiB
PHP
<?php
|
|
/**
|
|
* Plugin Name.
|
|
*
|
|
* @package Plugin_Name
|
|
* @author Your Name <email@example.com>
|
|
* @license GPL-2.0+
|
|
* @link http://example.com
|
|
* @copyright 2013 Your Name or Company Name
|
|
*/
|
|
|
|
/**
|
|
* Plugin class.
|
|
*
|
|
* TODO: Rename this class to a proper name for your plugin.
|
|
*
|
|
* @package Plugin_Name
|
|
* @author Your Name <email@example.com>
|
|
*/
|
|
class Plugin_Name {
|
|
|
|
/**
|
|
* Plugin version, used for cache-busting of style and script file references.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @const string
|
|
*/
|
|
const VERSION = '1.0.0';
|
|
|
|
/**
|
|
* Unique identifier for your plugin.
|
|
*
|
|
* Use this value (not the variable name) as the text domain when internationalizing strings of text. It should
|
|
* match the Text Domain file header in the main plugin file.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @var string
|
|
*/
|
|
protected $plugin_slug = 'plugin-name';
|
|
|
|
/**
|
|
* Instance of this class.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @var object
|
|
*/
|
|
protected static $instance = null;
|
|
|
|
/**
|
|
* Slug of the plugin screen.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @var string
|
|
*/
|
|
protected $plugin_screen_hook_suffix = null;
|
|
|
|
/**
|
|
* Initialize the plugin by setting localization, filters, and administration functions.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
private function __construct() {
|
|
|
|
// Load plugin text domain
|
|
add_action( 'init', array( $this, 'load_plugin_textdomain' ) );
|
|
|
|
// Add the options page and menu item.
|
|
// add_action( 'admin_menu', array( $this, 'add_plugin_admin_menu' ) );
|
|
|
|
// Add an action link pointing to the options page. TODO: Rename "plugin-name.php" to the name your plugin
|
|
// $plugin_basename = plugin_basename( plugin_dir_path( __FILE__ ) . 'plugin-name.php' );
|
|
// add_filter( 'plugin_action_links_' . $plugin_basename, array( $this, 'add_action_links' ) );
|
|
|
|
// Load admin style sheet and JavaScript.
|
|
add_action( 'admin_enqueue_scripts', array( $this, 'enqueue_admin_styles' ) );
|
|
add_action( 'admin_enqueue_scripts', array( $this, 'enqueue_admin_scripts' ) );
|
|
|
|
// Load public-facing style sheet and JavaScript.
|
|
add_action( 'wp_enqueue_scripts', array( $this, 'enqueue_styles' ) );
|
|
add_action( 'wp_enqueue_scripts', array( $this, 'enqueue_scripts' ) );
|
|
|
|
|
|
// Define custom functionality. Read more about actions and filters: http://codex.wordpress.org/Plugin_API#Hooks.2C_Actions_and_Filters
|
|
add_action( 'TODO', array( $this, 'action_method_name' ) );
|
|
add_filter( 'TODO', array( $this, 'filter_method_name' ) );
|
|
|
|
}
|
|
|
|
/**
|
|
* Return an instance of this class.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @return object A single instance of this class.
|
|
*/
|
|
public static function get_instance() {
|
|
|
|
// If the single instance hasn't been set, set it now.
|
|
if ( null == self::$instance ) {
|
|
self::$instance = new self;
|
|
}
|
|
|
|
return self::$instance;
|
|
}
|
|
|
|
/**
|
|
* Fired when the plugin is activated.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @param boolean $network_wide True if WPMU superadmin uses "Network Activate" action, false if WPMU is disabled or plugin is activated on an individual blog.
|
|
*/
|
|
public static function activate( $network_wide ) {
|
|
// TODO: Define activation functionality here
|
|
}
|
|
|
|
/**
|
|
* Fired when the plugin is deactivated.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @param boolean $network_wide True if WPMU superadmin uses "Network Deactivate" action, false if WPMU is disabled or plugin is deactivated on an individual blog.
|
|
*/
|
|
public static function deactivate( $network_wide ) {
|
|
// TODO: Define deactivation functionality here
|
|
}
|
|
|
|
/**
|
|
* Load the plugin text domain for translation.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function load_plugin_textdomain() {
|
|
|
|
$domain = $this->plugin_slug;
|
|
$locale = apply_filters( 'plugin_locale', get_locale(), $domain );
|
|
|
|
load_textdomain( $domain, trailingslashit( WP_LANG_DIR ) . $domain . '/' . $domain . '-' . $locale . '.mo' );
|
|
load_plugin_textdomain( $domain, FALSE, basename( dirname( __FILE__ ) ) . '/lang/' );
|
|
}
|
|
|
|
/**
|
|
* Register and enqueue admin-specific style sheet.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @return null Return early if no settings page is registered.
|
|
*/
|
|
public function enqueue_admin_styles() {
|
|
|
|
if ( ! isset( $this->plugin_screen_hook_suffix ) ) {
|
|
return;
|
|
}
|
|
|
|
$screen = get_current_screen();
|
|
if ( $screen->id == $this->plugin_screen_hook_suffix ) {
|
|
wp_enqueue_style( $this->plugin_slug .'-admin-styles', plugins_url( 'css/admin.css', __FILE__ ), array(), $this->version );
|
|
}
|
|
|
|
}
|
|
|
|
/**
|
|
* Register and enqueue admin-specific JavaScript.
|
|
*
|
|
* @since 1.0.0
|
|
*
|
|
* @return null Return early if no settings page is registered.
|
|
*/
|
|
public function enqueue_admin_scripts() {
|
|
|
|
if ( ! isset( $this->plugin_screen_hook_suffix ) ) {
|
|
return;
|
|
}
|
|
|
|
$screen = get_current_screen();
|
|
if ( $screen->id == $this->plugin_screen_hook_suffix ) {
|
|
wp_enqueue_script( $this->plugin_slug . '-admin-script', plugins_url( 'js/admin.js', __FILE__ ), array( 'jquery' ), $this->version );
|
|
}
|
|
|
|
}
|
|
|
|
/**
|
|
* Register and enqueue public-facing style sheet.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function enqueue_styles() {
|
|
wp_enqueue_style( $this->plugin_slug . '-plugin-styles', plugins_url( 'css/public.css', __FILE__ ), array(), $this->version );
|
|
}
|
|
|
|
/**
|
|
* Register and enqueues public-facing JavaScript files.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function enqueue_scripts() {
|
|
wp_enqueue_script( $this->plugin_slug . '-plugin-script', plugins_url( 'js/public.js', __FILE__ ), array( 'jquery' ), $this->version );
|
|
}
|
|
|
|
/**
|
|
* Register the administration menu for this plugin into the WordPress Dashboard menu.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function add_plugin_admin_menu() {
|
|
|
|
/*
|
|
* TODO:
|
|
*
|
|
* Change 'Page Title' to the title of your plugin admin page
|
|
* Change 'Menu Text' to the text for menu item for the plugin settings page
|
|
* Change 'plugin-name' to the name of your plugin
|
|
*/
|
|
$this->plugin_screen_hook_suffix = add_plugins_page(
|
|
__( 'Page Title', $this->plugin_slug ),
|
|
__( 'Menu Text', $this->plugin_slug ),
|
|
'read',
|
|
$this->plugin_slug,
|
|
array( $this, 'display_plugin_admin_page' )
|
|
);
|
|
|
|
}
|
|
|
|
/**
|
|
* Render the settings page for this plugin.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function display_plugin_admin_page() {
|
|
include_once( 'views/admin.php' );
|
|
}
|
|
|
|
/**
|
|
* Add settings action link to the plugins page.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function add_action_links( $links ) {
|
|
|
|
return array_merge(
|
|
array(
|
|
'settings' => '<a href="' . admin_url( 'plugins.php?page=plugin-name' ) . '">' . __( 'Settings', $this->plugin_slug ) . '</a>'
|
|
),
|
|
$links
|
|
);
|
|
|
|
}
|
|
|
|
/**
|
|
* NOTE: Actions are points in the execution of a page or process
|
|
* lifecycle that WordPress fires.
|
|
*
|
|
* WordPress Actions: http://codex.wordpress.org/Plugin_API#Actions
|
|
* Action Reference: http://codex.wordpress.org/Plugin_API/Action_Reference
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function action_method_name() {
|
|
// TODO: Define your action hook callback here
|
|
}
|
|
|
|
/**
|
|
* NOTE: Filters are points of execution in which WordPress modifies data
|
|
* before saving it or sending it to the browser.
|
|
*
|
|
* WordPress Filters: http://codex.wordpress.org/Plugin_API#Filters
|
|
* Filter Reference: http://codex.wordpress.org/Plugin_API/Filter_Reference
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function filter_method_name() {
|
|
// TODO: Define your filter hook callback here
|
|
}
|
|
|
|
}
|