mirror of
https://github.com/inretio/WordPress-Plugin-Boilerplate
synced 2025-02-27 20:05:57 +02:00
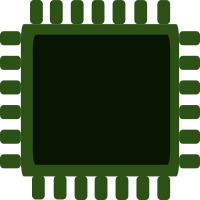
Also renaming the text domain in the header of the core plugin file to match that of the plugin name Fixes #194
215 lines
5.4 KiB
PHP
215 lines
5.4 KiB
PHP
<?php
|
|
|
|
/**
|
|
* The core plugin file
|
|
*
|
|
* This is the core plugin file that is used to define internationalization,
|
|
* dashboard-specific hooks, and public-facing site hooks.
|
|
*
|
|
* Also maintains the unique identifier of this plugin as well as the current
|
|
* version of the plugin.
|
|
*
|
|
* @link http://example.com
|
|
* @since 1.0.0
|
|
*
|
|
* @package Plugin_Name
|
|
* @subpackage Plugin_Name/includes
|
|
*/
|
|
|
|
/**
|
|
* Short Description. (use period)
|
|
*
|
|
* Long Description.
|
|
*
|
|
* @since 1.0.0
|
|
* @package Plugin_Name
|
|
* @subpackage Plugin_Name/includes
|
|
* @author Your Name <email@example.com>
|
|
*/
|
|
class Plugin_Name {
|
|
|
|
/**
|
|
* The loader that's responsible for maintaining and registering all hooks that power
|
|
* the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @access protected
|
|
* @var type Plugin_Name_Loader Maintains and registers all hooks for the plugin.
|
|
*/
|
|
protected $loader;
|
|
|
|
/**
|
|
* The unique identifier of this plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @access protected
|
|
* @var string $plugin_name The slug used to uniquely identify this plugin.
|
|
*/
|
|
protected $plugin_name;
|
|
|
|
/**
|
|
* The current version of the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @access protected
|
|
* @var string $version The current version of the plugin.
|
|
*/
|
|
protected $version;
|
|
|
|
/**
|
|
* Define the core functionality of the plugin.
|
|
*
|
|
* Set the plugin slug and the plugin version that can be used throughout the plugin.
|
|
* Load the dependencies, define the locale, and set the hooks for the Dashboard and
|
|
* the public-facing side of the site.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function __construct() {
|
|
|
|
$this->plugin_name = 'plugin-name';
|
|
$this->version = '1.0.0';
|
|
|
|
$this->load_dependencies();
|
|
$this->set_locale();
|
|
$this->define_admin_hooks();
|
|
$this->define_public_hooks();
|
|
|
|
}
|
|
|
|
/**
|
|
* Load the required dependencies for this plugin.
|
|
*
|
|
* Include the following files that make up the plugin:
|
|
*
|
|
* - Plugin_Name_Loader. Orchestrates the hooks of the plugin.
|
|
* - Plugin_Name_i18n. Defines internationalization functionality.
|
|
* - Plugin_Name_Admin. Defines all hooks for the dashboard.
|
|
* - Plugin_Name_Public. Defines all hooks for the public side of the site.
|
|
*
|
|
* Create an instance of the loader which will be used to register the hooks
|
|
* with WordPress.
|
|
*
|
|
* @since 1.0.0
|
|
* @access private
|
|
*/
|
|
private function load_dependencies() {
|
|
|
|
/**
|
|
* The class responsible for orchestrating the actions and filters of the
|
|
* core plugin.
|
|
*/
|
|
require_once plugin_dir_path( dirname( __FILE__ ) ) . 'includes/class-plugin-name-loader.php';
|
|
|
|
/**
|
|
* The class responsible for defining internationalization functionality
|
|
* of the plugin.
|
|
*/
|
|
require_once plugin_dir_path( dirname( __FILE__ ) ) . 'includes/class-plugin-name-i18n.php';
|
|
|
|
/**
|
|
* The class responsible for defining all actions that occur in the Dashboard.
|
|
*/
|
|
require_once plugin_dir_path( dirname( __FILE__ ) ) . 'admin/class-plugin-name-admin.php';
|
|
|
|
/**
|
|
* The class responsible for defining all actions that occur in the public-facing
|
|
* side of the site.
|
|
*/
|
|
require_once plugin_dir_path( dirname( __FILE__ ) ) . 'public/class-plugin-name-public.php';
|
|
|
|
$this->loader = new Plugin_Name_Loader();
|
|
|
|
}
|
|
|
|
/**
|
|
* Define the locale for this plugin for internationalization.
|
|
*
|
|
* Uses the Plugin_Name_i18n class in order to set the domain and to register the hook
|
|
* with WordPress.
|
|
*
|
|
* @since 1.0.0
|
|
* @access private
|
|
*/
|
|
private function set_locale() {
|
|
|
|
$plugin_i18n = new Plugin_Name_i18n();
|
|
$plugin_i18n->set_domain( $this->get_plugin_name() );
|
|
|
|
$this->loader->add_action( 'plugins_loaded', $plugin_i18n, 'load_plugin_textdomain' );
|
|
|
|
}
|
|
|
|
/**
|
|
* Register all of the hooks related to the dashboard functionality
|
|
* of the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @access private
|
|
*/
|
|
private function define_admin_hooks() {
|
|
|
|
$plugin_admin = new Plugin_Name_Admin( $this->get_plugin_name(), $this->get_version() );
|
|
|
|
$this->loader->add_action( 'admin_enqueue_scripts', $plugin_admin, 'enqueue_styles' );
|
|
$this->loader->add_action( 'admin_enqueue_scripts', $plugin_admin, 'enqueue_scripts' );
|
|
|
|
}
|
|
|
|
/**
|
|
* Register all of the hooks related to the public-facing functionality
|
|
* of the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @access private
|
|
*/
|
|
private function define_public_hooks() {
|
|
|
|
$plugin_public = new Plugin_Name_Public( $this->get_plugin_name(), $this->get_version() );
|
|
|
|
$this->loader->add_action( 'wp_enqueue_scripts', $plugin_public, 'enqueue_styles' );
|
|
$this->loader->add_action( 'wp_enqueue_scripts', $plugin_public, 'enqueue_scripts' );
|
|
|
|
}
|
|
|
|
/**
|
|
* Execute the loader to execute all of the hooks with WordPress.
|
|
*
|
|
* @since 1.0.0
|
|
*/
|
|
public function run() {
|
|
$this->loader->run();
|
|
}
|
|
|
|
/**
|
|
* The name of the plugin used to uniquely identify it within the context of
|
|
* WordPress and to define internationalization functionality.
|
|
*
|
|
* @since 1.0.0
|
|
* @return string The name of the plugin.
|
|
*/
|
|
public function get_plugin_name() {
|
|
return $this->plugin_name;
|
|
}
|
|
|
|
/**
|
|
* The reference to the class that orchestrates the hooks with the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @return Plugin_Name_Loader Orchestrates the hooks of the plugin.
|
|
*/
|
|
public function get_loader() {
|
|
return $this->loader;
|
|
}
|
|
|
|
/**
|
|
* Retrieve the version number of the plugin.
|
|
*
|
|
* @since 1.0.0
|
|
* @return string The version number of the plugin.
|
|
*/
|
|
public function get_version() {
|
|
return $this->version;
|
|
}
|
|
|
|
}
|